お疲れ様です。はるさらと申します。
今回は、シンプルなユーザー登録画面を、
HTMLとCSSを使って作成する方法を紹介します。
メールアドレスやパスワード、パスワード確認など、
一般的な入力項目を持つフォームを実装しており
コードも簡単に行いますので
ぜひお役立ていただければ幸いです。
ユーザー登録画面の基本構造
汎用的なユーザー登録画面は、
次の要素で構成されることが多いです。
- ユーザー名の入力欄
- メールアドレスの入力欄
- パスワードの入力欄
- パスワード確認欄
- 「登録」ボタン
- オプションとして、「すでにアカウントを持っていますか?」
のリンク見かけられます。
これらをもとに、HTMLでフォームを作成します。
完成すると下記のような
画面を作成することが出来ます。
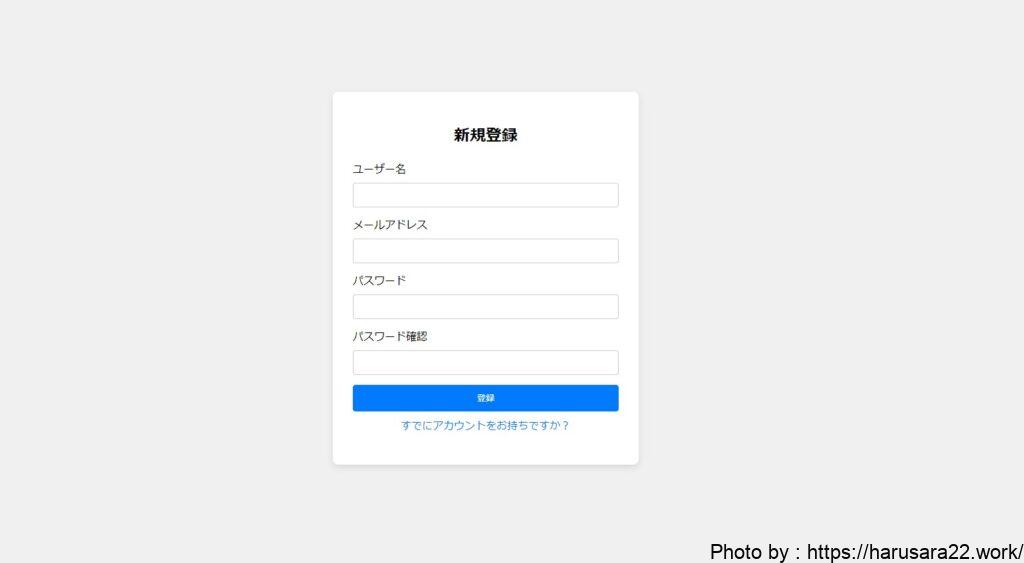
HTMLでユーザー登録フォームを作成
まず、基本的なHTML構造を記述します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | <!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>ユーザー登録</title> </head> <body> <div class="register-container"> <h2>新規登録</h2> <form action="/register" method="POST"> <div class="form-group"> <label for="username">ユーザー名</label> <input type="text" id="username" name="username" required> </div> <div class="form-group"> <label for="email">メールアドレス</label> <input type="email" id="email" name="email" required> </div> <div class="form-group"> <label for="password">パスワード</label> <input type="password" id="password" name="password" required> </div> <div class="form-group"> <label for="confirm-password">パスワード確認</label> <input type="password" id="confirm-password" name="confirm-password" required> </div> <button type="submit">登録</button> </form> <p><a href="#">すでにアカウントをお持ちですか?</a></p> </div> </body> </html> |
解説:
<form action="/register" method="POST">
:
フォームのデータをPOSTメソッドでサーバーに送信します。<input type="text">
:ユーザー名を入力するためのフィールド。<input type="email">
:
メールアドレス入力欄で、HTML5の検証機能を活用して
メールアドレス形式のチェックが可能です。<input type="password">
:
パスワード欄と確認欄を作成し、
2回入力を促すことで入力ミスを防ぎます。
CSSでスタイリング
次に、CSSを使って見た目を整え、
PC向けのデザインに仕上げます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | <style> body { font-family: Arial, sans-serif; background-color: #f0f0f0; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .register-container { background-color: white; padding: 30px; border-radius: 8px; box-shadow: 0 4px 10px rgba(0, 0, 0, 0.1); width: 400px; /* 固定幅を指定 */ text-align: center; } h2 { margin-bottom: 20px; } .form-group { margin-bottom: 15px; text-align: left; } label { display: block; margin-bottom: 8px; } input { width: 100%; padding: 10px; box-sizing: border-box; border: 1px solid #ccc; border-radius: 4px; } button { width: 100%; padding: 10px; background-color: #007bff; color: white; border: none; border-radius: 4px; cursor: pointer; } button:hover { background-color: #0056b3; } a { color: #007bff; text-decoration: none; } a:hover { text-decoration: underline; } p { margin-top: 10px; } </style> |
解説:
.register-container { width: 400px; }
:
PC向けに、横幅を400pxに固定しています。.form-group { margin-bottom: 15px; }
:
フォームの各入力欄に十分なスペースを持たせ、見やすく配置します。button:hover
: ホバー時にボタンの色を変え、
視覚的なフィードバックを提供します。
完成形のコード
以下が、PC向けのユーザー登録画面の完成形です。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 | <!DOCTYPE html> <html lang="ja"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>ユーザー登録</title> <style> body { font-family: Arial, sans-serif; background-color: #f0f0f0; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .register-container { background-color: white; padding: 30px; border-radius: 8px; box-shadow: 0 4px 10px rgba(0, 0, 0, 0.1); width: 400px; /* 固定幅を指定 */ text-align: center; } h2 { margin-bottom: 20px; } .form-group { margin-bottom: 15px; text-align: left; } label { display: block; margin-bottom: 8px; } input { width: 100%; padding: 10px; box-sizing: border-box; border: 1px solid #ccc; border-radius: 4px; } button { width: 100%; padding: 10px; background-color: #007bff; color: white; border: none; border-radius: 4px; cursor: pointer; } button:hover { background-color: #0056b3; } a { color: #007bff; text-decoration: none; } a:hover { text-decoration: underline; } p { margin-top: 10px; } </style> </head> <body> <div class="register-container"> <h2>新規登録</h2> <form action="/register" method="POST"> <div class="form-group"> <label for="username">ユーザー名</label> <input type="text" id="username" name="username" required> </div> <div class="form-group"> <label for="email">メールアドレス</label> <input type="email" id="email" name="email" required> </div> <div class="form-group"> <label for="password">パスワード</label> <input type="password" id="password" name="password" required> </div> <div class="form-group"> <label for="confirm-password">パスワード確認</label> <input type="password" id="confirm-password" name="confirm-password" required> </div> <button type="submit">登録</button> </form> <p><a href="#">すでにアカウントをお持ちですか?</a></p> </div> </body> </html> |
まとめ
今回は、基本的なHTMLフォームを使って
ユーザー登録画面を作成しましたが、
デザインを調整したり、
JavaScriptで入力チェックを追加することで、
さらに機能を追加していくことができます。
簡易的なシステムなモックの作成などに
お役立ていただければ幸いです。
どなたかのお役に立てれば幸いです。
それではまたー!